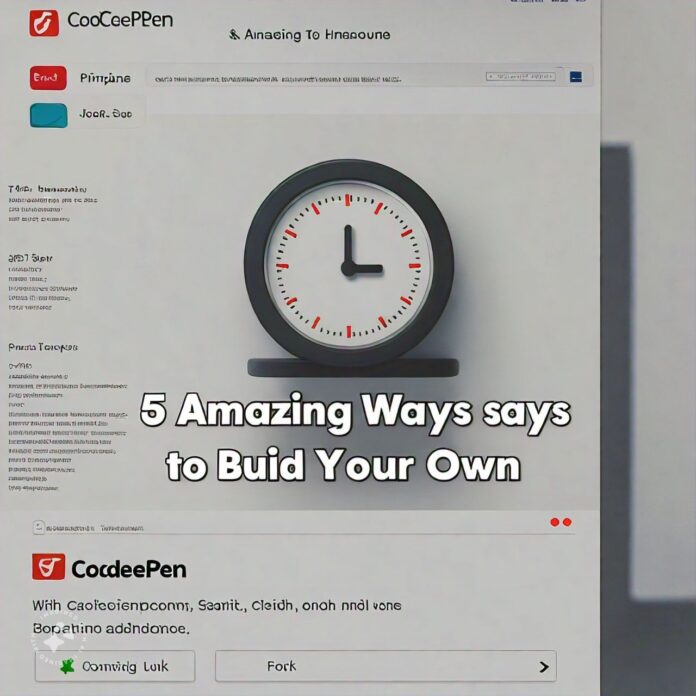
Introduction
Making intuitive web applications can be both tomfoolery and instructive, particularly while utilizing devices like CodePen and structures like ReactJS. In this article, we will investigate how to build a CodePen ReactJS timer. We’ll cover five amazing techniques that will help you comprehend the essentials of timers as well as further develop your ReactJS abilities. We should make a plunge!
What is CodePen?
Before we hop into our timer projects, how about we momentarily examine what CodePen is. CodePen is a web-based code proofreader and social improvement climate where you can compose code in the program and see the outcomes as you build. It upholds HTML, CSS, and JavaScript, making it an optimal stage for trying different things with ReactJS parts.
Why Use ReactJS for Building a Timer?
ReactJS is a strong JavaScript library for building UIs. It succeeds in making dynamic, single-page applications and is ideal for creating intuitive parts like timers. The decisive idea of Respond makes it simple to as needs be deal with the condition of our timer and update the UI.
1. Basic Countdown Timer
We should begin with a basic countdown timer. Here is an essential layout of how to make it:
Step-by-Step Guide:
Set Up CodePen: Open another pen on CodePen and add Respond and ReactDOM by means of the settings.
Create Timer Component:
const Timer = () => {
const [seconds, setSeconds] = React.useState(10);
React.useEffect(() => {
const interval = setInterval(() => {
setSeconds((prev) => (prev > 0 ? prev – 1 : 0));
}, 1000);
return () => clearInterval(interval);
}, []);
return <div>{seconds} seconds remaining</div>;
};
Render the Component:
ReactDOM.render(<Timer />, document.getElementById(‘root’));
2. Stopwatch with Start, Stop, and Reset
Next, let’s create a stopwatch that users can start, stop, and reset.
Step-by-Step Guide:
Create Stopwatch Component:
const Stopwatch = () => {
const [time, setTime] = React.useState(0);
const [running, setRunning] = React.useState(false);
React.useEffect(() => {
let interval = null;
if (running) {
interval = setInterval(() => {
setTime((prev) => prev + 1);
}, 1000);
} else if (!running && time !== 0) {
clearInterval(interval);
}
return () => clearInterval(interval);
}, [running, time]);
return (
<div>
<div>{time} seconds</div>
<button onClick={() => setRunning(true)}>Start</button>
<button onClick={() => setRunning(false)}>Stop</button>
<button onClick={() => setTime(0)}>Reset</button>
</div>
);
};
Render the Component as before.
3. Pomodoro Timer
The Pomodoro technique is an effective time management strategy. Let’s implement this next!
Step-by-Step Guide:
Create Pomodoro Timer Component:
const PomodoroTimer = () => {
const [time, setTime] = React.useState(25 * 60); // 25 minutes
const [isActive, setIsActive] = React.useState(false);
React.useEffect(() => {
let interval = null;
if (isActive && time > 0) {
interval = setInterval(() => {
setTime((prev) => prev – 1);
}, 1000);
} else if (!isActive && time !== 0) {
clearInterval(interval);
}
return () => clearInterval(interval);
}, [isActive, time]);
const minutes = Math.floor(time / 60);
const seconds = time % 60;
return (
<div>
{minutes}:{seconds < 10 ? `0${seconds}` : seconds}
<button onClick={() => setIsActive(true)}>Start</button>
<button onClick={() => setIsActive(false)}>Pause</button>
<button onClick={() => setTime(25 * 60)}>Reset</button>
</div>
);
};
Render the Component as usual.
4. Customizable Timer
A customizable timer allows users to set their desired time. Here’s how to do it.
Step-by-Step Guide:
const CustomTimer = () => {
const [inputTime, setInputTime] = React.useState(0);
const [time, setTime] = React.useState(0);
const [running, setRunning] = React.useState(false);
React.useEffect(() => {
let interval = null;
if (running && time > 0) {
interval = setInterval(() => {
setTime((prev) => prev – 1);
}, 1000);
} else if (!running && time !== 0) {
clearInterval(interval);
}
return () => clearInterval(interval);
}, [running, time]);
const handleStart = () => {
setTime(inputTime);
setRunning(true);
};
return (
<div>
<input
type=”number”
onChange={(e) => setInputTime(e.target.value)}
placeholder=”Set time in seconds”
/>
<button onClick={handleStart}>Start</button>
<div>{time} seconds remaining</div>
</div>
);
};
Render the Component.
5. Visual Timer
A visual timer can provide a more engaging user experience. Let’s create one!
Step-by-Step Guide:
Create Visual Timer Component:
const VisualTimer = () => {
const [time, setTime] = React.useState(60);
const [running, setRunning] = React.useState(false);
React.useEffect(() => {
let interval = null;
if (running && time > 0) {
interval = setInterval(() => {
setTime((prev) => prev – 1);
}, 1000);
} else if (!running) {
clearInterval(interval);
}
return () => clearInterval(interval);
}, [running, time]);
const percentage = (time / 60) * 100;
return (
<div>
<div style={{ width: `${percentage}%`, height: ’20px’, backgroundColor: ‘green’ }} />
<div>{time} seconds remaining</div>
<button onClick={() => setRunning(true)}>Start</button>
<button onClick={() => setRunning(false)}>Stop</button>
</div>
);
};
Render the Component.
Conclusion
Building a CodePen ReactJS timer can fundamentally upgrade how you might interpret Respond and JavaScript. Whether you pick a basic countdown, a stopwatch, or a visual timer, each venture offers one of a kind difficulties and learning potential open doors. Explore different avenues regarding these models on CodePen, and go ahead and change them to suit your requirements. Blissful coding!
FAQs about codepen reactjs timer
1. What is CodePen?
Reply: CodePen is a web-based code proofreader and social improvement climate where engineers can compose HTML, CSS, and JavaScript code in the program. It permits clients to explore, exhibit their work, and team up with others.
2. Why should I use ReactJS for building a timer?
Reply: ReactJS is a strong JavaScript library that works on the most common way of building dynamic UIs. Its part based engineering makes it simple to oversee state and update the UI, making it ideal for making intuitive parts like timers.
3. How do I create a basic countdown timer in ReactJS?
Reply: You can make a fundamental countdown timer by setting up a Respond part with state for the countdown worth and utilizing the useEffect snare to refresh it consistently. Really look at the article for a bit by bit guide.
4. What is the Pomodoro technique?
Reply: The Pomodoro strategy is a time usage technique that breaks work into spans, generally 25 minutes long, isolated by brief breaks. The article gives direction on the most proficient method to execute a Pomodoro timer utilizing ReactJS.
5. Can I customize the timer duration?
Reply: Yes! The adjustable timer project depicted in the article permits clients to enter their ideal time length. You can without much of a stretch change the timer to suit your inclinations.
6. How do I implement a visual timer?
Reply: To make a visual timer, you can utilize an advancement bar that tops off in view of the excess time. The article incorporates a bit by bit guide for building this component utilizing Respond.
7. Is it possible to add notifications to my timer?
Reply: Yes! You can add notices to your timer, like alarms when time is up. The article makes sense of how for carry out a countdown timer that sets off a ready when the time arrives at nothing.
8. What are some other timer projects I can try?
Reply: Other than the timers referenced in the article, you can explore different avenues regarding highlights like morning timers, stopwatches, or timers with extra functionalities like sound cautions or enhanced visualizations.
9. Do I need to install any software to use CodePen?
Reply: No, CodePen is an online stage, so you don’t have to introduce any product. You can begin coding squarely in your program.
10. Where can I find the example code from the article?
Reply: The model code scraps for every timer project are remembered for the article. You can reorder them into your CodePen climate to get everything rolling.
Go ahead and connect on the off chance that you have more inquiries or need further explanation!